Easy JavaScript AI Powered Tutorial for Beginners
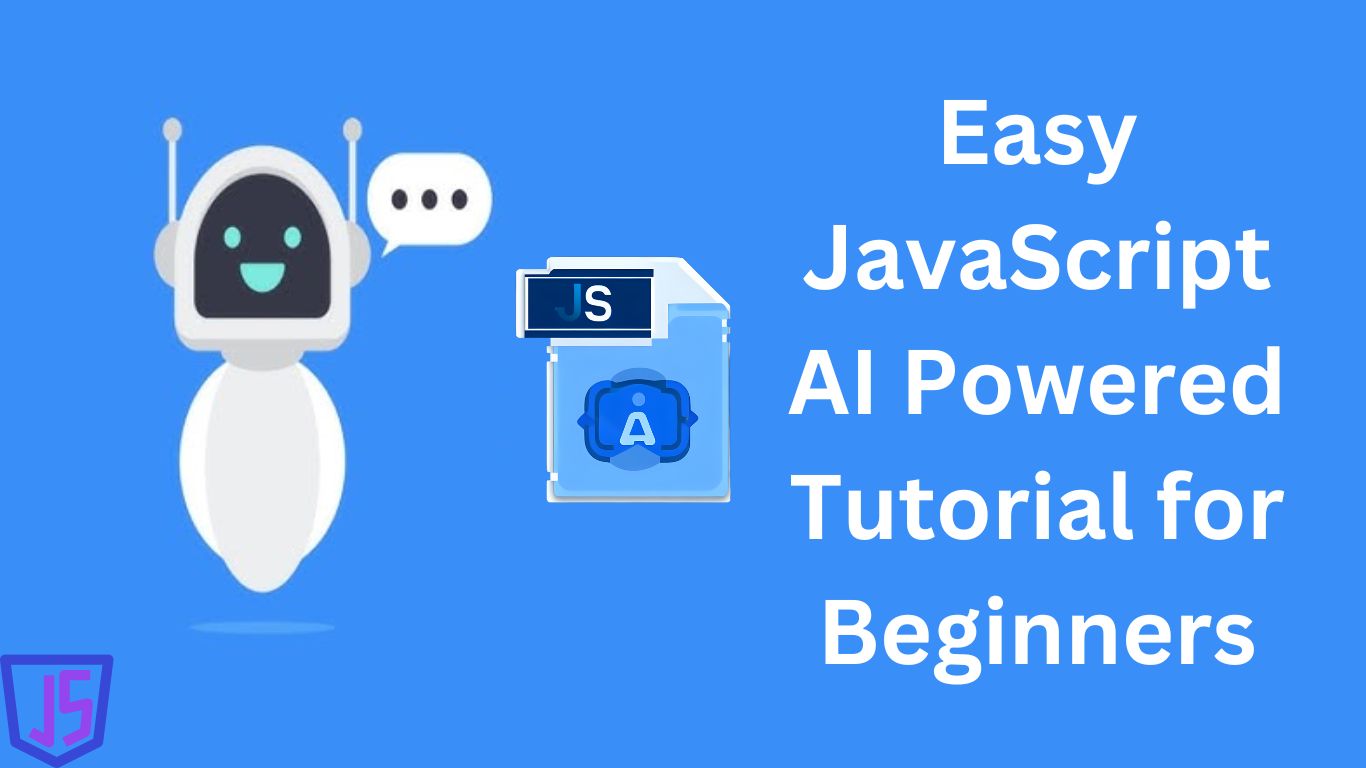
Welcome to an exciting journey into the world of JavaScript and Artificial Intelligence (AI)! Don't worry if you’re new to programming or AI; this guide is designed to be super simple and easy to understand, even if you’re just 10 years old. By the end of this tutorial, you’ll have a basic understanding of how to use JavaScript to create some cool AI-powered projects.
What is JavaScript?
JavaScript is a programming language that allows you to create interactive websites. If you’ve ever played a game online, clicked a button, or seen a moving image on a website, chances are JavaScript made that happen!
Why Learn JavaScript?
JavaScript is super popular because it’s used everywhere on the web. Learning JavaScript is like having a superpower that lets you build games, websites, and even control robots!
What is AI (Artificial Intelligence)?
Artificial Intelligence, or AI for short, is when computers are programmed to think and learn like humans. With AI, computers can recognize faces, understand speech, and even play games like chess or tic-tac-toe!
Combining JavaScript and AI
When you combine JavaScript with AI, you can create amazing things like smart chatbots that talk to you, games that learn from how you play, and much more. Let’s dive in and see how it’s done!
Setting Up Your Playground
Before we start, let’s set up a place where we can write and test our JavaScript code. You can do this online without installing anything!
Using Replit
One of the easiest ways to start coding in JavaScript is by using an online tool called Replit. Here’s how you can get started:
- Go to Replit.com.
- Click on “Start coding” and sign up if you haven’t already.
- Once signed in, click on the “+ New Repl” button.
- Select “JavaScript” from the list of languages.
- Name your project and click “Create Repl.”
Now you have a place to write and test your JavaScript code!
Your First JavaScript Program
Let’s start by writing a simple JavaScript program. We’re going to make the computer say "Hello, World!" This is a traditional first step when learning any programming language.
console.log("Hello, World!");
Type the code above into your Replit editor and click the “Run” button. You should see “Hello, World!” appear in the console below. Congratulations! You just wrote your first JavaScript program!

Making Your First AI with JavaScript
Now that you’ve written your first program, let’s move on to something more exciting: creating a simple AI that can guess a number!
Number Guessing Game
In this game, the computer will try to guess a number that you’re thinking of between 1 and 10. Here’s how to do it:
let numberToGuess = Math.floor(Math.random() * 10) + 1;
let userGuess = prompt("Guess a number between 1 and 10:");
if (parseInt(userGuess) === numberToGuess) {
console.log("Wow! You guessed it right! The number was " + numberToGuess);
} else {
console.log("Oops! The correct number was " + numberToGuess);
}
To run the use this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Guess the Number</title>
</head>
<body>
<h1>Guess the Number Game</h1>
<p id="result"></p> <!-- This is where the result will be displayed -->
<script>
// Generate a random number between 1 and 10
let numberToGuess = Math.floor(Math.random() * 10) + 1;
// Prompt the user to guess a number
let userGuess = prompt("Guess a number between 1 and 10:");
// Get the element where we will display the result
const resultElement = document.getElementById('result');
// Check if the user has input a valid number
if (userGuess !== null && !isNaN(userGuess)) {
if (parseInt(userGuess) === numberToGuess) {
resultElement.textContent = "Wow! You guessed it right! The number was " + numberToGuess;
} else {
resultElement.textContent = "Oops! The correct number was " + numberToGuess;
}
} else {
resultElement.textContent = "Invalid input. Please enter a number.";
}
</script>
</body>
</html>
Copy and paste the code above into your Replit editor and run it. The computer will pick a random number between 1 and 10, and you’ll have to guess what it is. If you guess correctly, it will tell you that you’re right!
Input Image of Number Guessing Game
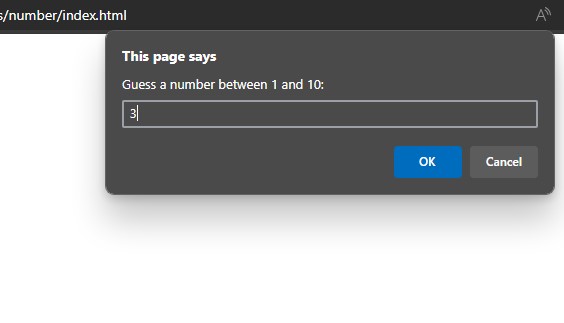
Output Image of Number Guessing Game
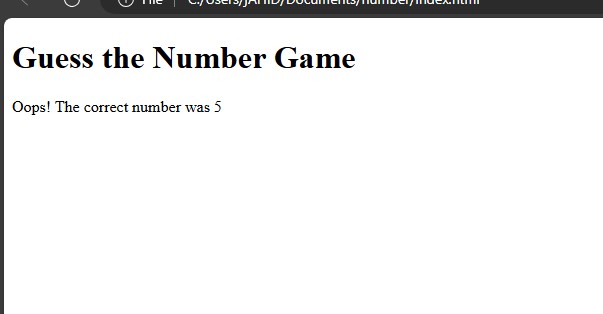
How Does It Work?
Let’s break down what’s happening in the code:
- Math.floor(Math.random() * 10) + 1: This code picks a random number between 1 and 10.
- prompt(): This asks the player to enter a number.
- if (parseInt(userGuess) === numberToGuess): This checks if the player’s guess is the same as the computer’s number.
Isn’t it cool how you can create a simple AI like this with just a few lines of JavaScript? Imagine what else you can do as you learn more!
What's Next?
Now that you’ve made a simple AI, the sky's the limit! Here are a few ideas for what you can try next:
- Create a chatbot that can talk to you.
- Make a game where the AI learns how to win.
- Build a website that reacts to what you say.
Frequently Asked Questions (FAQs)
1. Do I need to be good at math to learn JavaScript and AI?
No, you don't need to be a math whiz! While some math can help, you can still do a lot with JavaScript and AI by learning step by step.
2. Can I learn JavaScript on my own?
Yes, you can! There are plenty of free resources online, and with practice, you’ll get better and better.
3. What can I build with JavaScript?
You can build games, websites, interactive stories, and even control robots! The possibilities are endless.
4. Is AI difficult to learn?
It can be challenging, but by starting with simple projects like the one we did today, you can learn AI step by step.
5. Where can I practice more JavaScript?
You can practice on websites like Codecademy, freeCodeCamp, and of course, on Replit!
6. How long will it take to learn JavaScript and AI?
It depends on how much time you spend practicing. But remember, the key is to have fun while learning!
Conclusion
You’ve taken your first steps into the world of JavaScript and AI, and that’s something to be proud of! Remember to keep experimenting and building new projects. The more you practice, the better you’ll get. Who knows? Maybe one day you’ll create the next big AI invention!
Happy coding!